Rust 1.88.0 enhancements: Rust 1.88.0: A New Leap in Safe Programming!
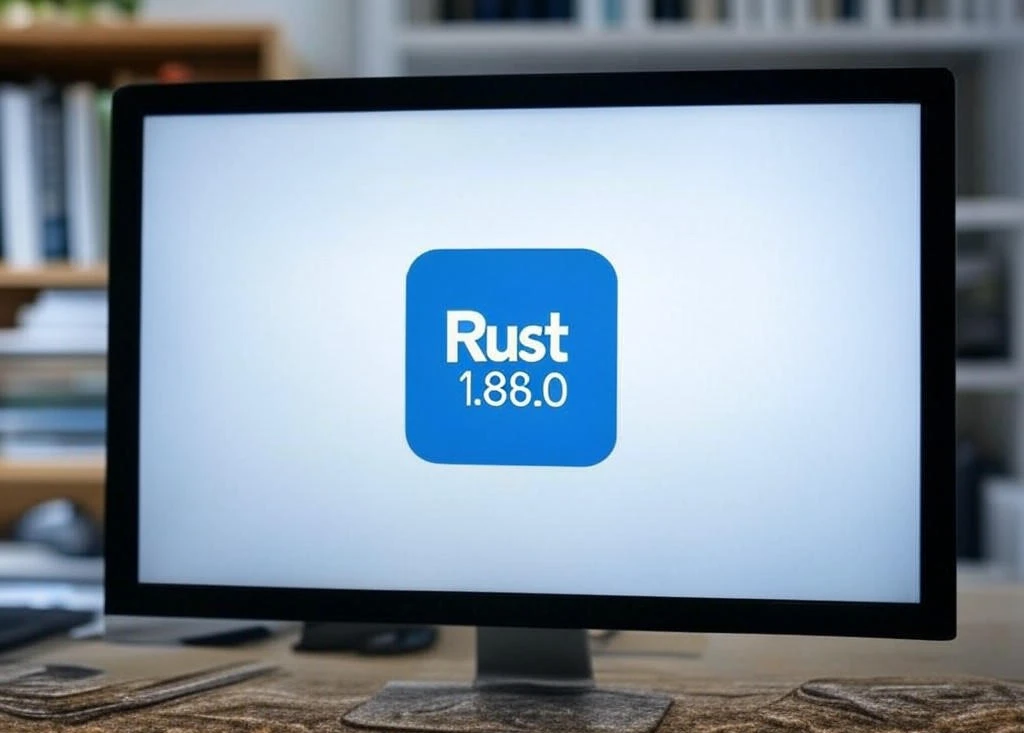
This website uses cookies
We use Cookies to ensure better performance, recognize your repeat visits and preferences, as well as to measure the effectiveness of campaigns and analyze traffic. For these reasons, we may share your site usage data with our analytics partners. Please, view our Cookie Policy to learn more about Cookies. By clicking «Allow all cookies», you consent to the use of ALL Cookies unless you disable them at any time.
Blockchain technology has emerged as a transformative force, reshaping industries, enhancing security, and revolutionizing how we transact and share data. Its decentralized and tamper-resistant nature holds the promise of creating a more transparent and trustable digital world. As blockchain's relevance continues to grow, so does the need for versatile tools that can harness its potential.
In this article, we embark on a journey to explore the symbiotic relationship between blockchain technology and Python, one of the most popular and versatile programming languages in the world. Python's role in blockchain development and interaction is significant and far-reaching, making it an invaluable tool for developers and businesses alike.
Blockchain technology, often hailed as the backbone of cryptocurrencies like Bitcoin, has transcended its origins to become a disruptive force across various industries.
At its core, blockchain is a distributed and immutable ledger that records transactions across a network of computers. These transactions are grouped into blocks, which are linked together in a chronological and secure chain. The term "blockchain" stems from this structure.
Decentralization: Unlike traditional centralized systems, blockchain operates on a decentralized network of computers (nodes). This decentralization eliminates the need for intermediaries, reducing costs and enhancing transparency.
Immutability: Once data is recorded on the blockchain, it becomes extremely difficult to alter or delete. This immutability ensures data integrity and trust.
Transparency: Every transaction on the blockchain is visible to all network participants. While the identities of users are typically pseudonymous, the transaction history is open for scrutiny.
Security: Blockchain employs cryptographic techniques to secure data and transactions. Consensus algorithms ensure that only valid transactions are added to the ledger.
Smart Contracts: Smart contracts are self-executing contracts with predefined rules and conditions. They automate processes and enable trustless interactions.
Blockchain technology has transcended its cryptocurrency origins to impact a wide range of industries:
Finance: Blockchain disrupts traditional financial systems with faster, cheaper, and more secure transactions. It enables borderless remittances, tokenized assets, and decentralized finance (DeFi) applications.
Supply Chain: Blockchain enhances transparency in supply chains, enabling real-time tracking of goods, verifying authenticity, and reducing fraud.
Healthcare: In healthcare, blockchain ensures the secure sharing of patient data, facilitates drug traceability, and enhances clinical trials' transparency.
Real Estate: Blockchain simplifies property transactions, reduces fraud, and increases transparency in real estate transactions.
Government: Governments explore blockchain for secure voting systems, identity verification, and tamper-proof record keeping.
Energy: Blockchain enables peer-to-peer energy trading, optimizing energy distribution and reducing costs.
Understanding the core principles and significance of blockchain technology lays the foundation for grasping how Python plays a crucial role in unlocking its potential.
Python has emerged as a powerhouse in the world of programming, known for its simplicity, readability, and extensive library ecosystem. When it comes to blockchain development, Python's popularity and versatility make it a standout choice.
Python has captured the hearts of developers worldwide, ranking consistently among the most popular programming languages. Its popularity stems from several key attributes:
Readability: Python's clean and concise syntax makes it easy for developers to write and understand code, facilitating rapid development.
Extensive Libraries: Python boasts a vast collection of libraries and frameworks that cover a wide spectrum of applications. This rich ecosystem accelerates development by providing pre-built solutions for various tasks.
Community Support: Python benefits from a robust and active developer community. This community-driven approach results in frequent updates, extensive documentation, and a wealth of resources for learning and troubleshooting.
Python's role in blockchain development is multifaceted, spanning several crucial areas:
Smart Contracts: Python is utilized in the creation of smart contracts, self-executing contracts with predefined rules and conditions. Smart contract platforms like Ethereum offer Solidity, a specialized language, but Python's simplicity and versatility make it a preferred choice for many developers.
Blockchain Protocols: Python enables the development of blockchain protocols and nodes. Developers use Python to create custom blockchains or modify existing ones to suit specific requirements.
Decentralized Applications (DApps): Python is employed in building decentralized applications that interact with blockchain networks. DApps can cover a wide range of domains, from finance to supply chain.
Blockchain Integration: Python facilitates the integration of blockchain functionality into existing applications and systems. This integration can enhance transparency, security, and data integrity.
Python offers several advantages when it comes to blockchain projects:
Rapid Development: Python's simplicity and extensive library support enable developers to prototype and develop blockchain solutions quickly.
Readability: Python code is easy to understand, reducing the likelihood of errors and simplifying collaboration among development teams.
Large Developer Pool: The abundance of Python developers ensures a broad talent pool to draw from when working on blockchain projects.
Cross-Platform Compatibility: Python is platform-agnostic, allowing developers to write code that runs on various operating systems.
Community and Documentation: The Python community provides excellent support, and extensive documentation and tutorials make it accessible to both beginners and experts.
As we delve deeper into this article, we will explore Python's role in developing blockchain applications, interacting with diverse blockchain networks, and the practical benefits it offers to developers and businesses in the blockchain space.
Python's adaptability and rich library ecosystem empower developers to create innovative blockchain applications efficiently.
Developing blockchain applications with Python involves a structured process:
Problem Identification: Begin by identifying the problem or use case your blockchain application aims to address. Understanding the requirements is crucial.
Design and Architecture: Create a design and architectural plan for your blockchain application. Define the data structure, consensus mechanism, and smart contract logic.
Development: Write the code for your blockchain application using Python. This phase includes developing smart contracts, creating the blockchain network, and integrating necessary features.
Testing: Rigorously test your application to ensure its functionality and security. This phase involves unit testing, integration testing, and security auditing.
Deployment: Deploy your blockchain application to the desired network or platform. Depending on your project, this could be a public blockchain network like Ethereum or a private, permissioned network.
Maintenance and Updates: Continuously monitor and maintain your application. Regular updates may be required to adapt to changing requirements or to address security vulnerabilities.
Python offers a range of libraries and frameworks that streamline blockchain development:
Web3.py: Web3.py is a Python library that allows developers to interact with Ethereum-based smart contracts and blockchain networks. It simplifies tasks like sending transactions, querying data, and managing accounts.
PySol: PySol provides a development environment for creating and testing Ethereum smart contracts in Python. It offers a convenient way to experiment with contract logic before deploying them to the blockchain.
Chaincode (Hyperledger Fabric): For building applications on Hyperledger Fabric, Python can be used as a scripting language within chaincode. This enables developers to leverage Python's capabilities in the context of Hyperledger Fabric networks.
Let's explore a couple of code examples and case studies to illustrate Python's role in blockchain application development:
# Python code to deploy a simple smart contract on Ethereum using Web3.py
from web3 import Web3
# Connect to an Ethereum node
w3 = Web3(Web3.HTTPProvider('https://infura.io/your-api-key'))
# Define the smart contract code
contract_code = """
pragma solidity ^0.8.0;
contract SimpleStorage {
uint256 public data;
function set(uint256 _data) public {
data = _data;
}
function get() public view returns (uint256) {
return data;
}
}
"""
# Deploy the smart contract
from solcx import compile_source
compiled_sol = compile_source(contract_code)
contract_interface = compiled_sol['<stdin>:SimpleStorage']
SimpleStorage = w3.eth.contract(
abi=contract_interface['abi'],
bytecode=contract_interface['bin']
)
# Deploy the contract
tx_hash = SimpleStorage.constructor().transact()
tx_receipt = w3.eth.waitForTransactionReceipt(tx_hash)
# Interact with the contract
simple_storage = w3.eth.contract(
address=tx_receipt.contractAddress,
abi=contract_interface['abi']
)
# Set and retrieve data
simple_storage.functions.set(42).transact({'from': w3.eth.accounts[0]})
stored_data = simple_storage.functions.get().call()
print(f"Stored data: {stored_data}")
A company uses Python to create a blockchain-based supply chain application. The application records product information on the blockchain at each stage of the supply chain, ensuring transparency and traceability. Smart contracts automatically trigger actions, such as payment releases, upon successful delivery, enhancing trust among stakeholders.
These examples and case studies demonstrate Python's utility in developing blockchain applications. In the following sections, we will explore Python's role in interacting with diverse blockchain platforms and delve into practical applications within specific industries.
Python's adaptability extends beyond blockchain development; it also serves as a versatile tool for interacting with diverse blockchain platforms.
Ethereum, the pioneer of smart contract platforms, is a prime candidate for Python-based interactions. Python libraries like Web3.py facilitate seamless communication with Ethereum's blockchain. Developers leverage Python for various Ethereum-related tasks, including:
Smart Contract Interaction: Python allows developers to deploy, call, and manage Ethereum smart contracts. This interaction is crucial for decentralized applications (DApps) that rely on smart contract logic.
Data Retrieval: Python scripts can retrieve blockchain data, including transaction history, token balances, and contract states. This information is invaluable for analytics and reporting.
Transaction Management: Python enables the creation and signing of Ethereum transactions, enabling users to send Ether, tokens, or interact with smart contracts programmatically.
Node Operations: Python can be used to manage Ethereum nodes, enabling developers to run and maintain their Ethereum infrastructure.
Binance Smart Chain, a blockchain platform compatible with the Ethereum Virtual Machine (EVM), has gained popularity for its lower transaction fees and scalability. Python's versatility seamlessly extends to BSC, allowing developers to:
Cross-Chain Compatibility: Python libraries like Web3.py can be adapted to interact with BSC nodes and smart contracts. This cross-chain compatibility enables developers to leverage Python skills for BSC projects.
Token Operations: Python scripts can facilitate token transfers, liquidity provision, and staking on BSC, supporting DeFi projects and token ecosystems.
DApp Development: Python developers can create DApps that run on BSC, offering users a wide range of decentralized services and utilities.
Interacting with blockchains via Python opens the door to various use cases:
Wallet Management: Python-based scripts can manage cryptocurrency wallets, facilitating secure storage and efficient asset management.
Oracles: Python can be used to create oracle services that fetch real-world data and feed it into smart contracts, enhancing their functionality.
DeFi Interactions: Python scripts can automate interactions with decentralized finance (DeFi) protocols, including liquidity provision, yield farming, and lending.
NFT Marketplaces: Python applications can be developed to manage NFT (Non-Fungible Token) marketplaces, allowing users to create, trade, and showcase digital assets.
Blockchain Analytics: Python-based tools enable the collection, analysis, and visualization of blockchain data, supporting insights into blockchain activities.
As Python continues to evolve and blockchain ecosystems expand, the possibilities for interaction and innovation are limitless. Python's role as a bridge to diverse blockchains empowers developers to explore and leverage the potential of these decentralized networks for a wide range of applications.
Smart contracts, self-executing agreements with predefined rules, have emerged as a cornerstone of blockchain technology. Python, known for its simplicity and versatility, plays a significant role in the creation and development of smart contracts.
While some blockchain platforms, like Ethereum, have their native smart contract languages (e.g., Solidity), Python's appeal has led to its integration as a scripting language for creating and interacting with smart contracts. Here's how Python contributes to the world of smart contracts:
Scripting Language: Python serves as a scripting language for Ethereum, allowing developers to write and execute smart contract logic in Python. This feature simplifies smart contract development, as Python is renowned for its ease of use and readability.
Prototyping and Testing: Python is an excellent choice for prototyping and testing smart contracts. Developers can quickly iterate through contract designs using Python before translating them into the platform's native language.
Enhanced Accessibility: Python's widespread adoption means that more developers are already familiar with the language, lowering the barrier to entry for smart contract development. This accessibility fosters innovation and attracts a broader developer community.
Ecosystem Integration: Python's extensive ecosystem of libraries and frameworks can be leveraged to support smart contract development. This includes tools for testing, security analysis, and deployment.
Python's role in smart contract development brings several advantages to the table:
Simplicity: Python's straightforward syntax makes smart contract code more accessible and easier to understand. This simplicity reduces the risk of errors and enhances code maintainability.
Rapid Development: Python's ease of use accelerates smart contract development, allowing developers to build, test, and deploy contracts quickly. This agility is essential in a fast-paced blockchain environment.
Readability: Python code is known for its readability, making it an ideal choice for smart contract development, where clarity and transparency are paramount.
Testing and Debugging: Python's extensive testing libraries and debugging tools simplify the identification and resolution of issues during the development process.
Versatility: Python's versatility extends to its use in oracles and off-chain components, allowing for seamless integration of external data sources and services with smart contracts.
As Python's role in the smart contract landscape continues to evolve, it offers developers an attractive alternative for creating and interacting with blockchain-based agreements. Whether you're a seasoned developer or new to blockchain, Python's presence in smart contract development empowers you to harness the transformative potential of blockchain technology.
While Python offers numerous advantages for blockchain development, it's essential to be aware of potential challenges and considerations when using Python in this context.
Challenge: Python is an interpreted language, which can result in slower execution speeds compared to lower-level languages like C++. In blockchain applications where efficiency is crucial, performance bottlenecks can be a concern.
Solution: Optimize critical sections of your code by using Python libraries like Cython to compile Python to machine code. Consider offloading computationally intensive tasks to lower-level languages or using specialized blockchain platforms designed for high throughput.
Challenge: Security is paramount in blockchain development, as vulnerabilities can lead to substantial financial losses or data breaches. Python's flexibility can inadvertently introduce vulnerabilities if not carefully managed.
Solution: Follow best practices for secure coding, such as input validation, authentication, and authorization. Leverage Python's extensive ecosystem of security libraries and tools for code analysis and vulnerability scanning. Perform thorough code audits and testing to identify and rectify security flaws.
Challenge: Ensuring cross-platform compatibility can be challenging, as different blockchain platforms and nodes may require adjustments to Python scripts.
Solution: Maintain compatibility by using libraries like Web3.py that abstract the intricacies of interacting with various blockchain networks. Regularly update your dependencies to stay current with platform changes.
Challenge: Python's blockchain libraries, while robust, may not offer the same level of native support as languages specifically designed for certain platforms (e.g., Solidity for Ethereum).
Solution: Explore third-party libraries and tools designed to enhance Python's capabilities in specific blockchain ecosystems. Collaborate with the community to develop and improve Python libraries for your chosen blockchain platform.
Challenge: Although Python is known for its simplicity, blockchain development involves complex concepts, particularly for newcomers to the field.
Solution: Invest time in learning both Python and blockchain fundamentals. Leverage online courses, tutorials, and blockchain developer communities to gain insights and knowledge. Start with smaller projects to build confidence and gradually tackle more complex tasks.
Challenge: As blockchain applications grow, scalability becomes a concern. Python's performance limitations may pose challenges when dealing with large-scale systems.
Solution: Plan for scalability from the beginning by designing your architecture to accommodate growth. Consider using microservices and optimizing critical components for scalability. Monitor system performance and be prepared to implement scaling solutions as needed.
Addressing these challenges and considerations proactively will help developers harness the full potential of Python in blockchain development. By staying informed, collaborating with the community, and continuously improving your skills, you can overcome obstacles and contribute to the ever-evolving blockchain ecosystem.
The world of blockchain development is dynamic and ever-evolving, and Python continues to play a pivotal role in shaping its future.
Emerging Trend: The integration of decentralized finance (DeFi) and Web3 technologies is gaining momentum. Python is well-positioned to facilitate the development of DeFi applications, smart contracts, and decentralized exchanges.
Opportunity: Developers skilled in Python can seize opportunities to create innovative DeFi solutions, such as lending platforms, yield farming protocols, and decentralized stablecoins. Python's versatility allows for the rapid prototyping of DeFi projects, contributing to the growth of this financial ecosystem.
Emerging Trend: The non-fungible token (NFT) market is expanding beyond digital art and collectibles. NFTs are being used for gaming assets, virtual real estate, intellectual property, and more.
Opportunity: Python developers can explore opportunities in building NFT marketplaces, minting platforms, and NFT-related tools. Python's readability and robust libraries make it an ideal choice for developing these NFT-centric applications and services.
Emerging Trend: As blockchain ecosystems continue to diversify, there is a growing need for interoperability solutions that allow different blockchains to communicate and share data seamlessly.
Opportunity: Developers proficient in Python can contribute to interoperability projects by creating bridges, cross-chain platforms, and middleware solutions. Python's adaptability is an asset in addressing the challenges of blockchain interoperability.
Emerging Trend: Privacy-focused blockchains and enhanced security measures are becoming a top priority. Solutions like zero-knowledge proofs (ZKPs) and privacy coins are gaining traction.
Opportunity: Python developers with expertise in cryptography and security can explore opportunities to contribute to privacy-centric blockchain projects. Python's libraries and tools facilitate the implementation of advanced security features.
Emerging Trend: With the increasing adoption of blockchain technology, the demand for education and training in this field is growing. This includes blockchain development courses, tutorials, and documentation.
Opportunity: Developers who are knowledgeable in Python and blockchain can take on roles as educators and trainers, sharing their expertise through online courses, workshops, and documentation. This not only contributes to the community but also offers opportunities for income and recognition.
Emerging Trend: Cross-chain solutions that enable assets and data to move seamlessly between different blockchains are gaining prominence.
Opportunity: Python developers can explore opportunities in cross-chain development by creating interoperable applications and solutions that bridge the gap between various blockchain networks. Python's versatility makes it a suitable choice for such projects.
In the dynamic landscape of blockchain development, staying updated with emerging trends and exploring new opportunities is essential. Python's adaptability, readability, and robust libraries position it as a valuable tool for developers to pioneer innovative blockchain solutions and contribute to the growth of this transformative technology.
As the blockchain space continues to evolve, Python developers have the chance to be at the forefront of groundbreaking projects that shape the future of decentralized systems and applications.
Real-world case studies provide valuable insights into the practical applications of Python-based blockchain projects.
Case Study Overview: Yearn Finance is a decentralized finance (DeFi) platform that automates yield farming strategies to maximize returns for users. It utilizes Python extensively in its development.
Python's Role: Python plays a crucial role in the creation of Yearn Finance's smart contracts and its backend infrastructure. The platform's smart contracts are written in Solidity (Ethereum's native language) but Python is used to interact with these contracts, automate strategies, and manage user funds.
Outcome: Yearn Finance has gained widespread recognition and adoption within the DeFi community. Its yield optimization strategies, implemented in Python, have consistently delivered competitive returns to users. Yearn Finance serves as an excellent example of Python's utility in DeFi project development.
Case Study Overview: Chainlink is a decentralized oracle network that connects smart contracts with real-world data sources and external APIs. Python plays a pivotal role in Chainlink's node development and ecosystem expansion.
Python's Role: Chainlink nodes, which facilitate data retrieval and communication between smart contracts and external sources, can be developed using Python. Chainlink's ecosystem includes Python-based tools for node operators and developers, enabling them to interact with the network seamlessly.
Outcome: Chainlink has become a fundamental component of the DeFi space, enabling smart contracts to access real-world data securely and reliably. Python's contributions to Chainlink's ecosystem have made it more accessible to a broader developer community.
Case Study Overview: Theta Network is a blockchain-based video streaming platform that aims to improve the streaming experience for both content creators and viewers. Python plays a role in developing the network's core functionalities.
Python's Role: Python is used in Theta Network's smart contract development, backend infrastructure, and analytics tools. It assists in managing content delivery, ensuring efficient video streaming, and optimizing network performance.
Outcome: Theta Network has gained popularity as a decentralized alternative to traditional video streaming platforms. Its use of Python in various aspects of development contributes to the network's scalability and user-friendliness.
Case Study Overview: Uniswap is a decentralized exchange (DEX) that allows users to swap various cryptocurrencies without the need for intermediaries. Python is employed in its development and analytics.
Python's Role: Python is used in Uniswap's smart contract interactions, backend services, and data analytics. It enables liquidity providers to manage their assets, execute trades, and access valuable market data.
Outcome: Uniswap has emerged as one of the leading decentralized exchanges in the DeFi space. Python's role in its development has contributed to its efficiency and user-friendliness, making it a preferred choice for decentralized trading.
These case studies exemplify the diverse applications of Python in blockchain projects, spanning DeFi, oracles, video streaming, and decentralized exchanges. Python's versatility, readability, and robust libraries have played pivotal roles in the success of these ventures. As the blockchain space continues to evolve, Python's role is likely to expand, offering developers new opportunities to innovate and contribute to the ecosystem.
In the dynamic and transformative world of blockchain technology, Python has emerged as a powerful ally for developers, offering versatility, simplicity, and a robust ecosystem of libraries and tools. This article has explored Python's essential role in blockchain development, from its contributions to smart contracts and blockchain interactions to its involvement in emerging trends and successful real-world projects.
As the blockchain landscape continues to evolve, Python remains a valuable tool for developers seeking to create transformative solutions and shape the future of decentralized systems. With its accessibility and adaptability, Python empowers both newcomers and experienced developers to explore and contribute to the limitless possibilities of blockchain technology.
In the ever-expanding universe of blockchain development, Python stands as a reliable companion, ready to facilitate innovation, overcome challenges, and unlock the full potential of this groundbreaking technology.
George Burlakov
2 min
LEARN MOREGeorge Burlakov
8 min
LEARN MOREGeorge Burlakov
8 min
LEARN MOREDmitriy Malets
10 min
LEARN MORE