Rust in Detail: Powerful Features That Simplify Developers' Lives
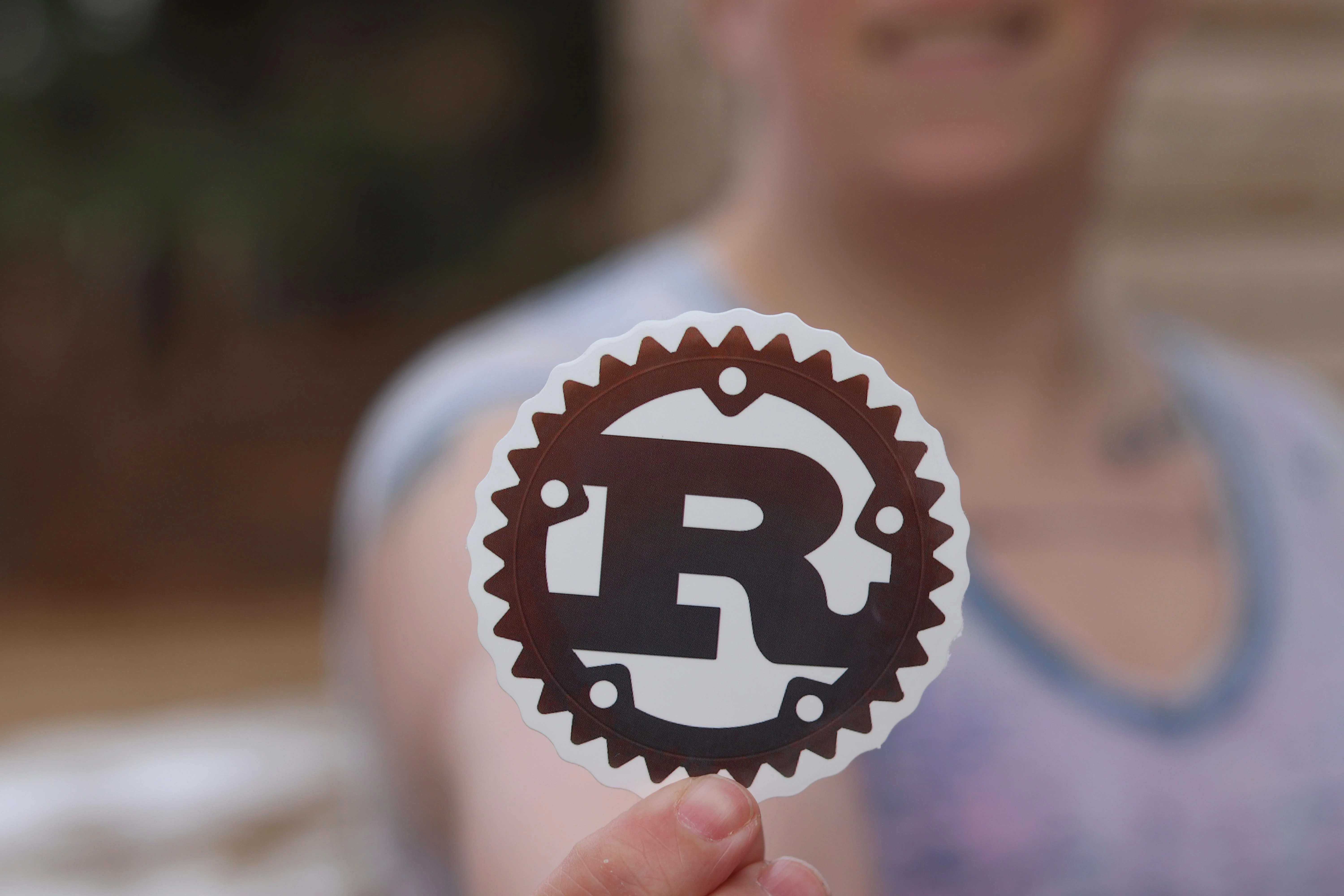
This website uses cookies
We use Cookies to ensure better performance, recognize your repeat visits and preferences, as well as to measure the effectiveness of campaigns and analyze traffic. For these reasons, we may share your site usage data with our analytics partners. Please, view our Cookie Policy to learn more about Cookies. By clicking «Allow all cookies», you consent to the use of ALL Cookies unless you disable them at any time.
Immutability refers to the property of data that cannot be changed after it has been created. In programming, immutable objects ensure that once a value is assigned, it remains constant throughout its lifetime. This eliminates the risk of unintentional modifications, providing a solid foundation for building predictable and reliable software systems. Immutability is a fundamental concept in functional programming but has also gained significant importance in modern languages like Rust, which combines functional and imperative paradigms to enhance software design.
Immutability plays a critical role in software development by addressing several challenges:
Eliminating Side Effects: Mutable states are a common source of bugs, as they can be inadvertently modified by different parts of the program. Immutability ensures that data remains consistent and unaltered, preventing such issues.
Thread Safety: In multithreaded applications, immutable data removes the need for complex synchronization mechanisms, as it cannot be altered concurrently, making it inherently safe to share across threads.
Enhanced Debugging and Testing: Immutable structures are easier to debug and test because their state is fixed, making it simpler to reproduce and trace issues.
Performance Optimization: While immutability may seem to introduce overhead due to data copying, modern techniques like structural sharing allow new versions of data to reuse existing structures, minimizing memory usage and improving performance.
Rust takes immutability to the next level by making variables immutable by default. This design choice encourages developers to write safer and more robust code. Key advantages of Rust’s approach to immutability include:
Compiler-Enforced Safety: Rust's compiler strictly enforces immutability, catching potential errors at compile time rather than runtime, reducing the likelihood of bugs.
Explicit Mutability: By requiring developers to explicitly declare mutable variables using the mut
keyword, Rust makes the intent to modify data clear, improving code readability and maintainability.
Seamless Integration with Performance: Rust leverages features like zero-cost abstractions and ownership to manage immutable data efficiently, ensuring high performance without sacrificing safety.
Advanced Libraries for Immutability: Rust’s ecosystem includes powerful libraries, such as im
and rpds
, which provide persistent and immutable data structures optimized for real-world use cases.
Immutability is not just a theoretical concept but a practical tool for building safer, faster, and more reliable software. Rust’s focus on immutability as a core principle empowers developers to embrace these benefits effortlessly, making it a preferred language for modern application development.
One of Rust's most distinctive features is that variables are immutable by default. This means that once a variable is initialized with a value, its state cannot be changed. This design choice helps developers avoid unintended side effects caused by data modification, promoting safer and more predictable code.
Example:
let x = 5;
// x = 6; // This will cause a compile-time error because `x` is immutable.
Immutability ensures that the value of x
remains consistent throughout its lifecycle, reducing the chances of bugs related to state changes.
mut
for Creating Mutable VariablesIf a variable needs to be mutable, Rust requires an explicit declaration using the mut
keyword. This approach makes the developer’s intention to modify a variable clear and ensures that immutability is the default behavior, not the exception.
Example:
let mut y = 10;
y = 15; // This is valid because `y` is declared as mutable.
println!("Mutable variable y: {}", y);
This explicit requirement to declare mutability improves code clarity and helps maintainers quickly understand which parts of the code can change state.
In Rust, references to variables are also immutable by default. This means you cannot modify the value through a reference unless it is explicitly declared mutable. Rust enforces strict rules around references to ensure memory safety.
Immutable Reference Example:
let z = 20;
let r = &z;
// *r = 30; // This will cause an error because `r` is an immutable reference.
println!("Immutable reference r: {}", r);
Mutable Reference Example:
let mut a = 25;
let b = &mut a; // Mutable reference to `a`
*b = 30; // Modify the value through the mutable reference
println!("Mutable reference b: {}", b);
Rust also prevents multiple mutable references at the same time, which eliminates data races in concurrent programs.
Immutability extends to data structures in Rust. When a structure is created without the mut
keyword, all its fields are immutable unless explicitly declared otherwise.
Example:
struct Point {
x: i32,
y: i32,
}
let point = Point { x: 5, y: 10 };
// point.x = 15; // Error: Fields of the `point` structure are immutable.
If a structure needs to have mutable fields, it must be declared as mutable:
let mut point = Point { x: 5, y: 10 };
point.x = 15; // Now this is valid because `point` is mutable.
println!("Updated Point: ({}, {})", point.x, point.y);
By defaulting to immutability and requiring explicit declarations for mutability, Rust provides a foundation for writing safer, clearer, and more robust programs. Whether it’s simple variables, references, or complex data structures, Rust’s focus on immutability ensures a predictable and error-free coding experience, making it a standout choice for developers focused on performance and reliability.
The "Builder" pattern is a design pattern that facilitates the construction of complex objects step-by-step. In Rust, this pattern is particularly useful for managing immutability. It allows an object to be modified during its creation while ensuring that the final product is immutable.
Key benefits of the "Builder" pattern in Rust:
Safe Mutability During Creation: Allows temporary mutability for configuring an object while maintaining immutability once the object is finalized.
Readable and Chainable Syntax: Enables method chaining for a cleaner and more intuitive API.
Error Prevention: Minimizes the risk of accidental mutations after the object is constructed.
Here is an example of implementing the "Builder" pattern to create a Rectangle
structure:
#[derive(Debug)]
struct Rectangle {
width: u32,
height: u32,
}
impl Rectangle {
// Constructor to create a new Rectangle
fn new() -> Rectangle {
Rectangle { width: 0, height: 0 }
}
// Method to set the width, returning a mutable reference to allow chaining
fn set_width(&mut self, width: u32) -> &mut Rectangle {
self.width = width;
self
}
// Method to set the height, also returning a mutable reference
fn set_height(&mut self, height: u32) -> &mut Rectangle {
self.height = height;
self
}
// Finalizing method to "build" the immutable Rectangle
fn build(self) -> Rectangle {
self
}
}
fn main() {
// Using the Builder pattern to create a Rectangle
let rect = Rectangle::new()
.set_width(10)
.set_height(20)
.build();
println!("Final Rectangle: {:?}", rect);
// The object `rect` is now immutable
// rect.width = 15; // This will cause a compile-time error
}
Temporary Mutability: During the setup phase, methods like set_width
and set_height
operate on a mutable reference to modify the object's fields.
Finalization: The build
method consumes the mutable instance and returns a new immutable instance of the object.
Immutability After Construction: Once the object is constructed using the build
method, it becomes immutable, preventing further changes.
The "Builder" pattern in Rust elegantly handles the need for mutability during object creation while ensuring immutability afterward. This approach aligns perfectly with Rust’s philosophy of safety and predictability, making it a practical tool for creating complex, immutable data structures.
Immutability is crucial for building reliable and thread-safe systems. Rust supports immutability with both its native features and powerful external libraries like im
and rpds
, which provide persistent data structures. These structures allow modifications by creating new versions of data while sharing unmodified parts to optimize memory usage.
im
LibraryThe im
library provides persistent collections like immutable vectors and linked lists, which allow updates without modifying the original data.
use im::Vector;
fn main() {
let vec = Vector::new(); // Create an empty immutable vector
let updated_vec = vec.push_back(42); // Add an element to the vector
println!("Original vector: {:?}", vec);
println!("Updated vector: {:?}", updated_vec);
}
The vec
remains unchanged, while updated_vec
includes the added element.
This behavior is achieved through structural sharing, minimizing memory usage.
use im::conslist::ConsList;
fn main() {
let list = ConsList::new(); // Create an empty list
let list = list.cons(1).cons(2).cons(3); // Add elements
println!("Immutable List: {:?}", list);
}
Each call to cons
creates a new list with a reference to the previous one.
Structural sharing is a technique that reuses unmodified parts of a data structure when creating a new version. This avoids deep copying and enhances performance.
use rpds::Vector;
fn main() {
let vec1 = Vector::new().push_back(10).push_back(20); // Original vector
let vec2 = vec1.push_back(30); // New vector shares structure with vec1
println!("Original vector: {:?}", vec1);
println!("Updated vector: {:?}", vec2);
}
vec2
reuses most of vec1
’s data, and only the additional element is stored separately.
Hash maps and trees in im
and rpds
support immutability, allowing updates without modifying the original structure.
use im::HashMap;
fn main() {
let mut map = HashMap::new(); // Create an empty map
map = map.update("key1", "value1"); // Add a key-value pair
let map2 = map.update("key2", "value2"); // Create a new map with an additional pair
println!("Original map: {:?}", map);
println!("Updated map: {:?}", map2);
}
The original map
remains unchanged, while map2
includes the new key-value pair.
use im::OrdMap;
fn main() {
let tree = OrdMap::new(); // Create an empty ordered map (tree)
let tree = tree.update(1, "a").update(2, "b"); // Add elements
let tree2 = tree.update(3, "c"); // Create a new tree with an additional element
println!("Original tree: {:?}", tree);
println!("Updated tree: {:?}", tree2);
}
Trees ensure order while maintaining immutability and structural sharing.
im
and rpds
LibrariesUsing im
Library for Persistent Collections:
use im::Vector;
fn main() {
let vec = Vector::new();
let vec1 = vec.push_back(100);
let vec2 = vec1.push_back(200);
println!("Vec1: {:?}", vec1);
println!("Vec2: {:?}", vec2);
}
Using rpds
Library for Hash Maps:
use rpds::HashTrieMap;
fn main() {
let map = HashTrieMap::new();
let map1 = map.insert("key1", "value1");
let map2 = map1.insert("key2", "value2");
println!("Map1: {:?}", map1);
println!("Map2: {:?}", map2);
}
Immutable data structures in Rust, supported by libraries like im
and rpds
, offer powerful tools for building robust applications. By leveraging structural sharing, they ensure high performance while preserving immutability, making them ideal for concurrent and functional programming scenarios. These libraries empower developers to create efficient and predictable systems with minimal overhead.
Immutability is not just a theoretical concept; it plays a pivotal role in solving real-world challenges in software development. From managing state in multithreaded environments to functional programming paradigms, immutability enhances safety, predictability, and efficiency.
In multithreaded programs, shared mutable state is a common source of bugs like data races. Immutability eliminates these issues by ensuring that state cannot be modified after it is created. By sharing immutable data across threads, developers can achieve thread safety without requiring complex synchronization mechanisms.
Immutable data can be freely shared between threads without synchronization.
Guarantees that no thread can accidentally alter shared state.
Simplifies reasoning about program behavior.
Arc
for Safe AccessRust's Arc
(Atomic Reference Counting) is a smart pointer that enables multiple threads to share ownership of immutable data safely.
use std::sync::Arc;
use std::thread;
#[derive(Debug)]
struct Config {
api_url: String,
timeout: u32,
}
fn main() {
let config = Arc::new(Config {
api_url: "https://example.com".to_string(),
timeout: 30,
});
let threads: Vec<_> = (1..=3)
.map(|i| {
let config_clone = Arc::clone(&config);
thread::spawn(move || {
println!("Thread {}: Config - {:?}", i, config_clone);
})
})
.collect();
for thread in threads {
thread.join().unwrap();
}
}
Arc
ensures safe shared access to the immutable Config
object.
Each thread reads the same Config
without fear of race conditions.
Functional programming (FP) embraces immutability as a core principle. Rust allows developers to use immutable state to build predictable, maintainable systems. By updating state through pure functions, FP avoids side effects, making code easier to test and reason about.
In this example, we create a user state and update it immutably, returning a new version each time.
#[derive(Debug, Clone)]
struct UserState {
user_id: u32,
preferences: Vec<String>,
}
impl UserState {
fn add_preference(&self, preference: String) -> Self {
let mut new_preferences = self.preferences.clone();
new_preferences.push(preference);
UserState {
user_id: self.user_id,
preferences: new_preferences,
}
}
}
fn main() {
let state = UserState {
user_id: 1,
preferences: vec!["dark_mode".to_string()],
};
let updated_state = state.add_preference("notifications".to_string());
println!("Original State: {:?}", state);
println!("Updated State: {:?}", updated_state);
}
Each call to add_preference
creates a new UserState
with updated preferences.
The original state remains unchanged, ensuring immutability.
Immutability is a practical and powerful tool in Rust for addressing challenges in multithreaded programming and functional programming. By sharing immutable data across threads and updating state immutably, developers can create robust and predictable systems. Rust's features like Arc
and its efficient data management make immutability a natural fit for modern software development.
Rust’s ecosystem provides powerful libraries like im
and rpds
for working with immutable and persistent data structures. These libraries help developers manage state efficiently and safely, supporting functional programming paradigms and multithreaded applications.
im
LibraryThe im
library offers a rich set of persistent (immutable) data structures, including:
Vectors: Efficient immutable arrays.
ConsLists: Linked lists optimized for immutability.
HashMaps: Immutable key-value stores.
OrdMaps: Immutable ordered maps.
HashSets/OrdSets: Immutable sets for storing unique values.
Key Features:
Optimized for structural sharing to minimize memory overhead.
Allows easy creation and manipulation of immutable data.
When to Use: Ideal for applications requiring frequent state updates while preserving previous states, such as undo/redo functionality or functional programming.
rpds
LibraryThe rpds
library provides high-performance immutable data structures such as:
Vector: Persistent vectors with structural sharing.
HashTrieMap: Persistent hash maps.
HashTrieSet: Persistent hash sets.
Key Features:
High efficiency for large data sets.
Designed for safe use in concurrent or functional programming contexts.
When to Use: Best for applications with a focus on performance and scalability, such as real-time systems or large-scale computations.
im
Libraryuse im::Vector;
fn main() {
let vec = Vector::new(); // Create an empty vector
let vec1 = vec.push_back(10).push_back(20); // Add elements
let vec2 = vec1.push_back(30); // Create a new vector with additional elements
println!("Original Vector: {:?}", vec1);
println!("Updated Vector: {:?}", vec2);
}
vec1
remains unchanged while vec2
includes the new element, demonstrating structural sharing.
use im::HashMap;
fn main() {
let map = HashMap::new(); // Create an empty map
let map1 = map.update("key1", "value1"); // Add a key-value pair
let map2 = map1.update("key2", "value2"); // Create a new map with another pair
println!("Original Map: {:?}", map1);
println!("Updated Map: {:?}", map2);
}
Each update
creates a new map, preserving the original.
rpds
Libraryuse rpds::Vector;
fn main() {
let vec = Vector::new(); // Create an empty persistent vector
let vec1 = vec.push_back(100).push_back(200); // Add elements
let vec2 = vec1.push_back(300); // Create a new vector
println!("Original Vector: {:?}", vec1);
println!("Updated Vector: {:?}", vec2);
}
vec2
shares the structure with vec1
, adding only the new element.
use rpds::HashTrieMap;
fn main() {
let map = HashTrieMap::new(); // Create an empty hash map
let map1 = map.insert("key1", "value1"); // Add a key-value pair
let map2 = map1.insert("key2", "value2"); // Add another pair
println!("Map 1: {:?}", map1);
println!("Map 2: {:?}", map2);
}
map2
is a new version, while map1
remains unchanged.
The im
and rpds
libraries are indispensable tools for managing immutable data in Rust. They enable developers to work with persistent structures efficiently, leveraging immutability to build safer and more reliable applications. Whether you’re designing functional programs, multithreaded systems, or stateful applications, these libraries simplify handling state transitions while maintaining high performance.
Immutability is more than just a programming principle; it’s a practical tool that enhances the quality, safety, and maintainability of software. By working with immutable data structures, developers gain significant advantages in managing state, testing, debugging, and understanding their code.
Managing application state can be challenging, especially in systems with complex workflows or concurrency. Immutability simplifies this process by ensuring that data remains constant once created, leading to:
Predictable State Transitions: Every modification results in a new state, making changes explicit and easy to track.
Undo/Redo Functionality: Immutable structures naturally support maintaining a history of states, allowing seamless implementation of features like undo/redo in applications.
Reduced Complexity: Without mutable state, developers don’t need to account for the cascading effects of unintended data modifications.
#[derive(Debug, Clone)]
struct AppState {
count: u32,
}
impl AppState {
fn increment(&self) -> Self {
Self {
count: self.count + 1,
}
}
}
fn main() {
let state = AppState { count: 0 };
let new_state = state.increment();
println!("Original State: {:?}", state);
println!("Updated State: {:?}", new_state);
}
The original state
remains unchanged, making it easy to maintain and debug.
Immutability eliminates many sources of errors by ensuring data cannot be modified unexpectedly. This is particularly beneficial in:
Testing: Since immutable data is predictable, tests can focus on verifying outputs without worrying about intermediate state changes.
Multithreading: Immutable data can be shared safely across threads without needing locks or synchronization, preventing race conditions and deadlocks.
use std::sync::Arc;
use std::thread;
fn main() {
let data = Arc::new(vec![1, 2, 3]);
let handles: Vec<_> = (0..3)
.map(|_| {
let data_clone = Arc::clone(&data);
thread::spawn(move || {
println!("Shared Data: {:?}", data_clone);
})
})
.collect();
for handle in handles {
handle.join().unwrap();
}
}
The data remains immutable, ensuring thread safety without additional synchronization.
Immutability promotes clearer and more predictable code by:
Explicit Intent: Developers know that immutable variables cannot change, reducing cognitive load and making the code easier to understand.
Fewer Side Effects: Functions operating on immutable data are less prone to unintended consequences, resulting in code that behaves as expected.
Improved Debugging: Immutable data makes it easier to reproduce bugs, as the state of the application is deterministic and doesn’t change unexpectedly.
fn double_numbers(numbers: &Vec<i32>) -> Vec<i32> {
numbers.iter().map(|x| x * 2).collect()
}
fn main() {
let original = vec![1, 2, 3];
let doubled = double_numbers(&original);
println!("Original: {:?}", original);
println!("Doubled: {:?}", doubled);
}
The original vector remains unmodified, ensuring clear and predictable behavior.
Immutability transforms state management, testing, and code readability into strengths rather than challenges. By preventing unintended changes, eliminating race conditions, and promoting clarity, immutability helps developers create robust, maintainable, and scalable systems. Rust’s focus on immutability, combined with its strong type system and ownership model, makes it an ideal language for leveraging these advantages in modern software development.
Immutability is a foundational principle that enhances the safety, performance, and maintainability of software. In Rust, immutability is deeply integrated into the language, providing developers with powerful tools to:
Prevent unintended data modifications and eliminate side effects.
Simplify state management by ensuring predictable transitions.
Enable thread-safe sharing of data without the need for complex synchronization mechanisms.
Improve code readability and maintainability by making the intent explicit.
Facilitate testing and debugging by ensuring deterministic program behavior.
Rust’s approach to immutability, supported by its robust type system and ownership model, empowers developers to write reliable and efficient code that adheres to modern software development standards.
At Technorely, we specialize in Rust and understand how to unlock its full potential for your projects. Whether you're looking to implement immutability best practices, optimize your codebase, or build high-performance applications, we’re here to help.
Our team has extensive experience in developing scalable, secure, and efficient solutions with Rust, tailored to meet your unique needs. If you’re ready to elevate your project to new heights, simply fill out the Contact Us Form, and let’s collaborate to create something extraordinary together!
George Burlakov
8 min
LEARN MOREGeorge Burlakov
8 min
LEARN MOREGeorge Burlakov
5 min
LEARN MOREGeorge Burlakov
8 min
LEARN MORE